In this post, we will see how we can display variables from JavaScript in our Html document.
In JavaScript we can display text or any variable of Javascript in our HTML page using:
- Using
document.write()
method - Using
innerHTML
property, and - Using
window.alert()
method.
Table of Contents
Let’s understand each of the methods to display Javascript value with examples.
Display JavaScript variable using document.write() method
The document.write()
method is used to display text on an HTML page.
To display text we can use this.
document.write('Hello Everyone')
To display HTML code we can write like this.
document.write("<h2>Hello Everyone</h2>")
Next, we write this inside our <script>
tag to see the result.
<body>
<h1>Hello World</h1>
<p>Lorem ipsum dolor sitsum, nam!</p>
<script>
document.write('Hello Everyone');
document.write("<h2>Hello Everyone</h2>")
</script>
</body>
The output will be like this
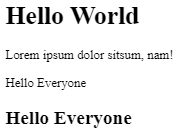
However, if we use document.write()
after a document is loaded, it will replace all the content inside the HTML <body>
tags with the HTML text and Javascript that is given in the document.write() method.
For example, let’s say we have a web-page with this content inside it and a function that runs when the page is loaded.
<body onload="myFunction()">
<h1>Hello World</h1>
<p>Lorem ipsum dolor sitsum, nam!</p>
<script>
function myFunction() {
document.write("<h2>Content Change</h2>");
}
</script>
</body>
This will replace all the content of the page with the one inside the document.write()
method.
Output:
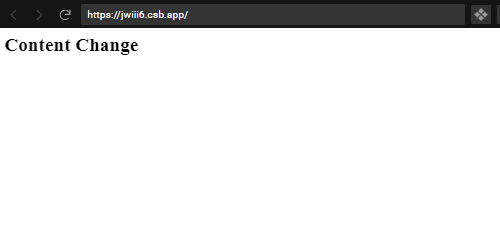
Display JavaScript variable using innerHTML property
The innerHTML
property in JavaScript is used to set or get/return HTML content of an element.
Suppose we have a div element in our web-page with an id as ‘header’.
<body>
<div id="myText">Hello World</div>
</body>
So to replace the ‘Hello World‘ text with a JavaScript variable name, first we have to target the element using its Id.
Using document.getElementById()
method, we target the element and then change the text with a variable value.
let newName = 'Welcome Everyone'
document.getElementById("myText").innerHTML = newName;
This will now change the value inside the HTML element with the new variable value, ‘Welcome Everyone
<body>
<div id="myText">Welcome Everyone</div>
</body>
Display JavaScript Variable using window.alert() method
The window.alert()
method is used to display a dialog box with text messages on our browsers.
The dialog box prevents the user from accessing the rest of the page until the dialog box is closed by the user using the ‘OK’ button.
We can use this method to show JavaScript variable values in our browsers.
Let’s see how we can pass a variable value in our window.alert() dialog box with an example.
<body>
<div onclick="showValue()">Display Variable value</div>
</body>
In our script tag
<script>
let textMsg = "Hello! I am a Box"
function showValue(){
window.alert(textMsg)
}
</script>
So when we click the button it shows the variable value in the alert dialog box.

Displaying the Javascript variable value as an output is a very common task in web-development. And with the help of JavaScript API, we can perform these actions very easily as it helps to manipulate our HTML elements.
And among the three methods discussed above, the most common way to display text on a webpage is by using the innerHTML
property. And the other two methods are also used but mainly for testing purposes.