In this tutorial, we will learn how we can trigger a button click function on pressing the enter button in an input field.
Let’s suppose we have a page with a form to input our name.
<template>
<div id="app">
<form>
<input v-model="name" placeholder="Enter your Name">
<button type="submit" @click="submitForm()">Submit</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
name: ""
};
},
methods: {
submitForm(e) {
e.preventDefault();
alert(this.name);
}
}
};
</script>
Here, in the above code, we have a form with an input field for name and a button to submit the form.
We have added a click event on the button which gets triggered when we click the submit button.
However, here in this tutorial, we want to submit the form by pressing Enter without clicking the submit button.
Let’s see how to do it below.
Submit by pressing Enter using the key modifiers in Vue
To trigger the click event on pressing Enter we have to use the key modifiers provided by Vue Js.
The key modifiers
are just event modifiers but these modifiers can be used with keyboard events.
For our example, we will use the @keyup.enter
modifier inside the input field of the form.
<template>
<div id="app">
<form>
<input v-model="name" placeholder="Enter your Name"
@keyup.enter = "submitForm">
<button type="submit" @click="submitForm">Submit</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
name: ""
};
},
methods: {
submitForm(e) {
e.preventDefault();
alert('Pressed Button',this.name);
},
}
};
</script>
We have added the keyup event with the enter modifier to trigger the function submitForm().
The enter
modifier ensures that the function is only executed when the user releases the enter key.
Now you can press the Enter key to trigger the button click or just use the Submit button to trigger the submitForm() function.
DEMO:
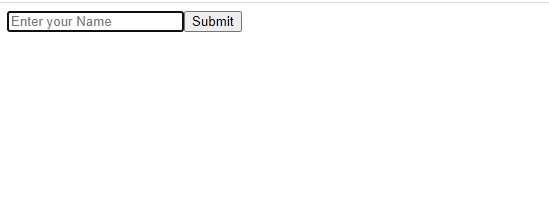