In this article, we will learn how to reference static image assets in Vue JS.
Let’s say we want to use an image that is saved locally in our assets folder in Vue project.
Table of Contents
To use it with an < img >
tags we can reference the image in two ways:
Using the relative path in Vue template
If you want to use it directly in Vue template we can do it like this.
<img alt="Vue logo" src="./assets/photo.jpeg" width="80%" />
We have directly used the image from the assets folder in our vue template.
Here we are using the relative path "./assets/photo.jpeg"
, it will get replaced by an auto-generated URL based on the Webpack output configuration.
Using require() to get image asset path
To get the assets path using JavaScript we use the require()
in Vue. This leverages the Webpack’s module and the file is processed by the file-loader and returns us the resolved URL.
<template>
<div id="app">
<img :src="img" width="80%" />
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
img: require("./assets/photo.jpeg"),
};
},
};
</script>
Here, we have used require() to reference the image in our data property, and using v-bind ( :src) we have bind it to the < img >
tag src attribute.
We can also use require() directly in our template but we have to bind it to the src attribute like this
<img alt="Vue logo" :src="require('./assets/photo.jpeg')" width="80%" />
DEMO:
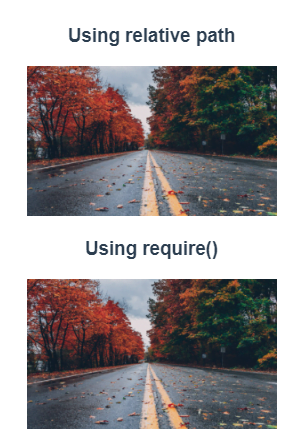